Learning Python for Beginners — Part 3
By Data Impala
In the last post, we explored how Python handles variables, data types, and simple operations.
You now know how to store information, do basic math, and write clear code.
But now it’s time to make your code smarter.
This article introduces two very important tools:
- Conditional logic — how to ask questions in code
- Loops — how to repeat tasks efficiently
You’ll use these again and again in real-world projects, whether you’re cleaning spreadsheets, analyzing numbers, or building apps.
Table of Contents
What is Conditional Logic?
Conditional logic means making decisions in your code.
Imagine this in real life:
If it’s raining, you carry an umbrella.
If you’re hungry, you eat.
If your phone battery is low, you charge it.
Python uses similar “if this, then that” logic to decide what to do based on certain conditions.
The if Statement
Here’s the basic structure:
if condition:
# Do something
Example:
temperature = 30
if temperature > 25:
print("It's a hot day!")
This checks if the number stored in temperature is more than 25.
If yes, it runs the print statement.
Adding else — What If the Condition Isn’t Met?
You can tell Python what to do if the condition is not true:
temperature = 20
if temperature > 25:
print("It's a hot day!")
else:
print("It's a cool day.")
Output:
It's a cool day.
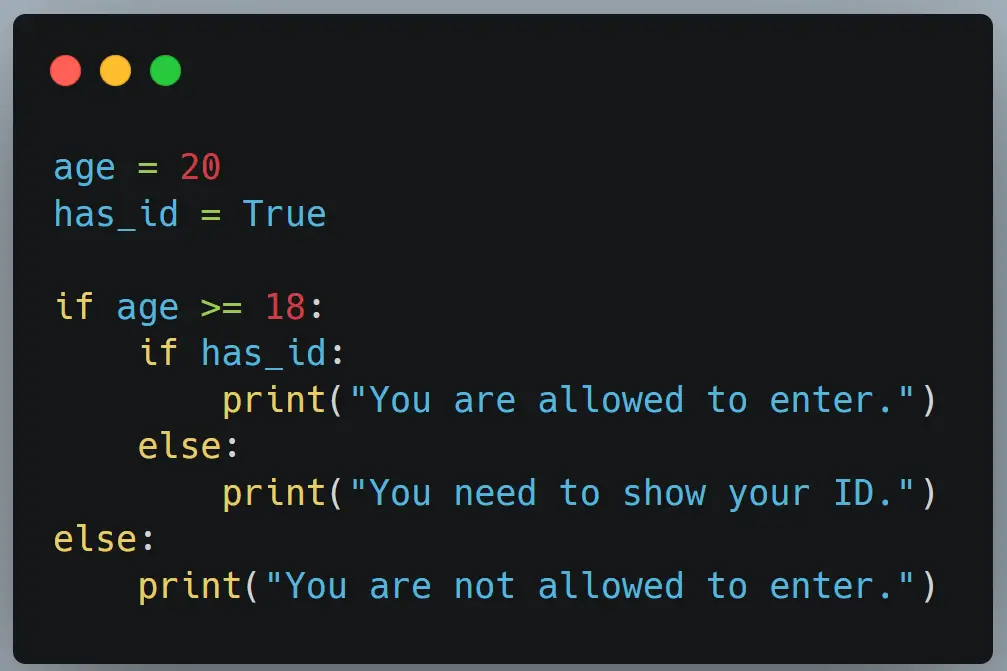
Using elif for Multiple Checks
Sometimes, you want to test several possibilities:
score = 85
if score >= 90:
print("Excellent!")
elif score >= 70:
print("Good job!")
else:
print("Keep practicing.")
Python checks from top to bottom.
Once a condition is true, it skips the rest.
Indentation Matters
Python doesn’t use curly braces or symbols to group blocks of code. It uses indentation — that means spacing.
So make sure to indent your code under if, else, or elif, usually with 4 spaces.
if True:
print("Correct") # This is indented, so it's inside the if block
Logical Operators
Sometimes you want to check more than one condition.
and – Both must be true
or – At least one must be true
not – Reverses the condition
Example:
age = 18
has_id = True
if age >= 18 and has_id:
print("Entry allowed")
Loops: Doing Things Again and Again
Now imagine you want to print the numbers from 1 to 10. You could write:
print(1)
print(2)
print(3)
...
But that’s painful and not very clever.
That’s where loops come in.
The while Loop
This repeats a block of code as long as a condition is true.
Example:
count = 1
while count <= 5:
print(count)
count += 1 # Adds 1 to count
This prints:
1
2
3
4
5
Once count becomes 6, the loop stops.
The for Loop
This is used to loop over a sequence (like a list or a range of numbers).
Example:
for number in range(1, 6):
print(number)
This gives the same result:
1
2
3
4
5
range(1, 6) gives the numbers from 1 up to (but not including) 6.
Loop Control: break, continue, and pass
break
Stops the loop early.
for i in range(1, 10):
if i == 5:
break
print(i)
This prints:
1
2
3
4
As soon as i is 5, the loop exits.
continue
Skips the current loop and jumps to the next round.
for i in range(1, 6):
if i == 3:
continue
print(i)
Output:
1
2
4
5
It skips 3 but continues with the rest.
pass
This does nothing. It’s a placeholder.
for i in range(5):
pass # Placeholder if you're not ready to write the code yet
Useful when you’re planning your structure but haven’t filled it in yet.
Let’s Build a Small Project Together
Here’s a fun example using both logic and loops.
Problem: You want to ask a user to enter a password. They get 3 tries.
correct_password = "impala123"
attempt = 0
max_attempts = 3
while attempt < max_attempts:
guess = input("Enter password: ")
if guess == correct_password:
print("Access granted")
break
else:
print("Wrong password.")
attempt += 1
else:
print("Too many tries. Locked out.")
This is how you combine loops, conditions, and inputs to build something useful.
Recap
You’ve just learned how to control how your Python code behaves, and that’s a big deal.
Here’s what you now know:
- How to write if, else, and elif statements
- How to check conditions using and, or, and not
- How to write while and for loops
- How to control loops using break, continue, and pass
What’s Coming Up Next?
Now that you know how to make decisions and repeat actions, the next step is learning how to work with multiple values at once using lists, tuples, sets, and dictionaries — Python’s built-in data containers.
These tools will let you build apps that can handle collections of data — from shopping lists to survey results.
Stay tuned for Part 4 of the series on “Working with Lists, Tuples, and Dictionaries in Python.”
Written by Ahnaf Chowdhury
Series: Learning Python for Beginners — Part 3